介绍
覆盖和重载是Java编程中的核心概念。它们是在我们的Java程序中实现多态性的方式。多态性是面向对象编程的概念之一。面向对象编程概念
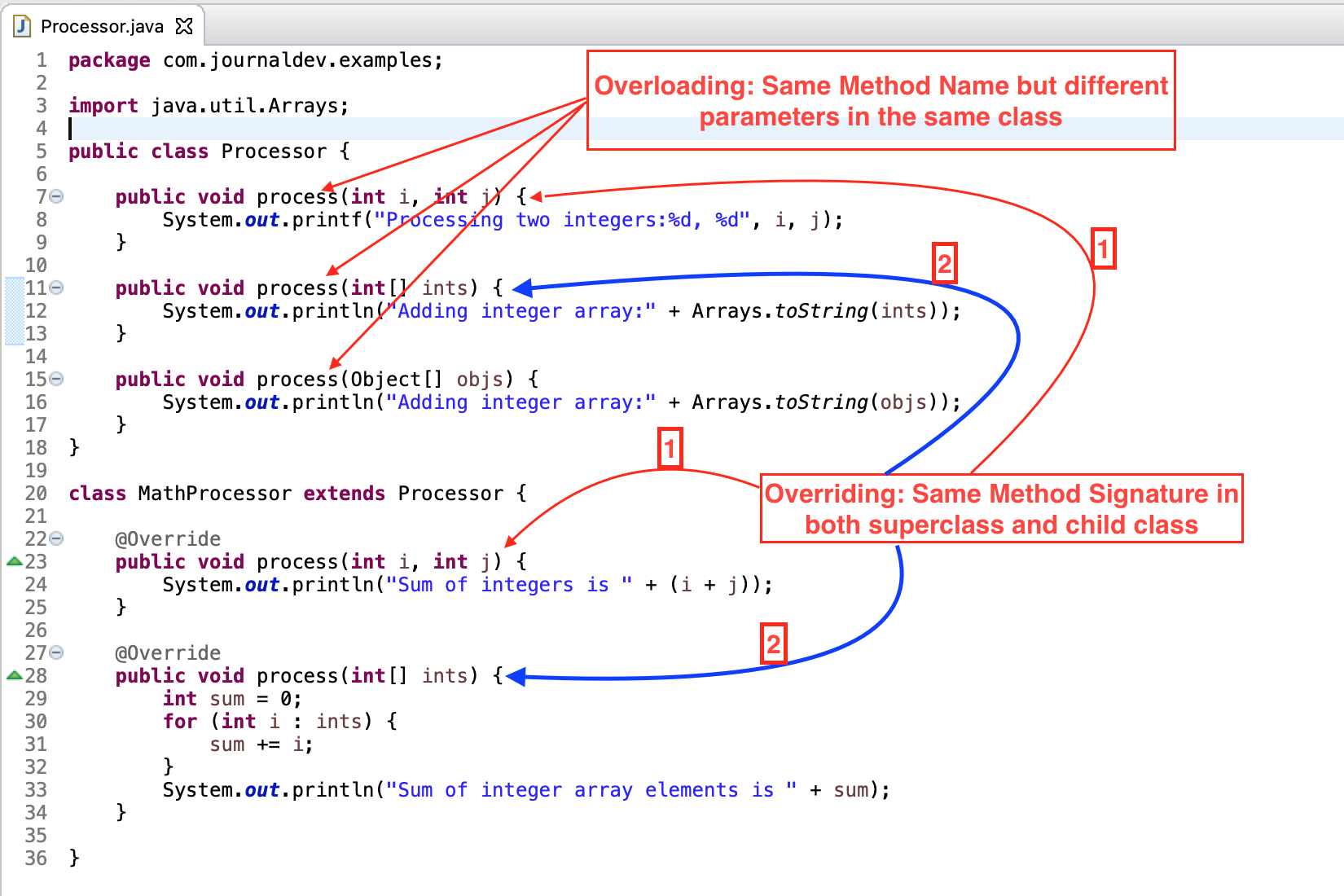
当在超类和子类中方法签名(名称和参数)相同时,称为覆盖。当同一类中的两个或更多方法具有相同的名称但具有不同的参数时,称为重载。
比较覆盖和重载
Overriding | Overloading |
---|---|
Implements “runtime polymorphism” | Implements “compile time polymorphism” |
The method call is determined at runtime based on the object type | The method call is determined at compile time |
Occurs between superclass and subclass | Occurs between the methods in the same class |
Have the same signature (name and method arguments) | Have the same name, but the parameters are different |
On error, the effect will be visible at runtime | On error, it can be caught at compile time |
覆盖和重载示例
以下是Java程序中覆盖和重载的示例:
package com.journaldev.examples;
import java.util.Arrays;
public class Processor {
public void process(int i, int j) {
System.out.printf("Processing two integers:%d, %d", i, j);
}
public void process(int[] ints) {
System.out.println("Adding integer array:" + Arrays.toString(ints));
}
public void process(Object[] objs) {
System.out.println("Adding integer array:" + Arrays.toString(objs));
}
}
class MathProcessor extends Processor {
@Override
public void process(int i, int j) {
System.out.println("Sum of integers is " + (i + j));
}
@Override
public void process(int[] ints) {
int sum = 0;
for (int i : ints) {
sum += i;
}
System.out.println("Sum of integer array elements is " + sum);
}
}
覆盖
process()
方法和 int i, int j
参数在 Processor
类中被子类 MathProcessor
覆盖。第 7 行和第 23 行:
public class Processor {
public void process(int i, int j) { /* ... */ }
}
/* ... */
class MathProcessor extends Processor {
@Override
public void process(int i, int j) { /* ... */ }
}
而在 Processor
类中,process()
方法和 int[] ints
也被子类覆盖。第 11 行和第 28 行:
public class Processor {
public void process(int[] ints) { /* ... */ }
}
/* ... */
class MathProcessor extends Processor {
@Override
public void process(Object[] objs) { /* ... */ }
}
重载
process()
方法在 Processor
类中被重载。第 7、11 和 15 行:
public class Processor {
public void process(int i, int j) { /* ... */ }
public void process(int[] ints) { /* ... */ }
public void process(Object[] objs) { /* ... */ }
}
结论
在这篇文章中,我们涵盖了Java中的重写(overriding)和重载(overloading)。当在超类和子类中的方法签名相同时,就会发生重写。当同一类中的两个或更多方法具有相同的名称但参数不同时,就会发生重载。
Source:
https://www.digitalocean.com/community/tutorials/overriding-vs-overloading-in-java