KotlinはJetBrainsからの最新のJVMプログラミング言語です。Googleはこれを公式のAndroid開発言語とし、Javaと共に採用しています。開発者たちはJavaプログラミングで直面する問題に対処すると言います。私は多くのKotlinチュートリアルを書いており、ここでは重要なKotlin面接の質問を提供しています。
Kotlinの面接の質問
ここでは、Kotlinの面接の質問と回答を提供しています。これらのKotlinの面接の質問は初心者だけでなく経験豊富なプログラマにも役立ちます。コーディングのスキルを向上させるためのコーディングの質問もあります。
-
Kotlinのターゲットプラットフォームは何ですか? Kotlin-Javaの相互運用性はどのように実現されていますか?
Java Virtual Machine(JVM)はKotlinのターゲットプラットフォームです。KotlinはJavaと完全に互換性があり、どちらもコンパイルするとバイトコードが生成されます。したがって、KotlinコードはJavaから呼び出すことができ、逆もまた然りです。
-
Kotlinで変数を宣言する方法は?宣言方法はJavaとどう違いますか?
JavaとKotlinの変数宣言には2つの主な違いがあります:
-
宣言の型 Javaでは、宣言は次のようになります:
String s = "Java String"; int x = 10;
Kotlinでは、宣言は次のようになります:
val s: String = "Hi" var x = 5
Kotlinでは、宣言は
val
またはvar
で始まり、オプションの型が続きます。Kotlinは型推論を使用して型を自動的に検出できます。 -
デフォルト値 Javaでは次のように可能です:
String s:
Kotlinの次の変数宣言は無効です。
val s: String
-
-
valとvar宣言の違いは何ですか?StringをIntに変換するにはどうすればいいですか?
val
変数は変更できません。これらはJavaのfinal修飾子のようです。var
は再代入可能で、再代入する値は同じデータ型でなければなりません。fun main(args: Array<String>) { val s: String = "Hi" var x = 5 x = "6".toInt() }
StringをIntに変換するには、
toInt()
メソッドを使用します。 -
Null SafetyとNullable TypesはKotlinで何ですか?Elvis Operatorは何ですか?
KotlinはNull Pointer Exceptionsを防ぐアプローチとしてnull safetyに注力しており、これは
String?
、Int?
、Float?
などのnullable typesを使用しています。これらはラッパータイプとして機能し、nullの値を保持できます。nullableな値は他のnullableまたは基本的な型の値に追加できません。基本的な型を取得するには、Nullable Typesをアンラップするセーフコールを使用する必要があります。アンラップ時に値がnullである場合、無視するかデフォルト値を代わりに使用できます。Elvis OperatorはNullableから値を安全にアンラップするために使用されます。これはnullableタイプの上に?:
として表されます。右側の値はnullableタイプがnullを保持している場合に使用されます。var str: String? = "JournalDev.com" var newStr = str?: "Default Value" str = null newStr = str?: "Default Value"
-
「const」とは何ですか?それは「val」とどう違いますか?
デフォルトでは、
val
プロパティは実行時に設定されます。val
にconst修飾子を追加すると、コンパイル時定数になります。const
はvar
と単独では使用できません。const
はローカル変数には適用できません。 -
Kotlinはint、float、doubleなどのプリミティブ型を使用できますか?
言語レベルでは、上記の型を使用することはできません。ただし、コンパイルされたJVMバイトコードには確かに存在します。
-
すべてのKotlinプログラムのエントリーポイントは何ですか?
main
関数は、すべてのKotlinプログラムのエントリーポイントです。Kotlinでは、クラス内にmain関数を書かないことも選択することができます。JVMにコンパイルすると、暗黙的にクラスにカプセル化されます。Array<String>
形式で渡される文字列は、コマンドライン引数を取得するために使用されます。 -
!!
は、null可能な値を強制的にアンラップして値を取得するために使用されます。返される値がnullの場合、ランタイムクラッシュが発生します。したがって、!!
演算子は値が絶対にnullにならないことを完全に確信している場合にのみ使用する必要があります。それ以外の場合は、望ましくないnullポインター例外が発生します。一方、?.はElvis演算子で、安全な呼び出しを行います。null可能な値に安全にアンラップするために、以下に示すようにラムダ式let
を使用できます。ここで、let式はnull可能な型を安全にアンラップします。
!!
は、null可能な型を強制的にアンラップして値を取得するために使用されます。返される値がnullの場合、ランタイムクラッシュが発生します。したがって、!!
演算子は値が絶対にnullにならないことを完全に確信している場合にのみ使用する必要があります。それ以外の場合は、望ましくないnullポインター例外が発生します。一方、?.はElvis演算子で、安全な呼び出しを行います。null可能な値に安全にアンラップするために、以下に示すようにラムダ式let
を使用できます。ここで、let式はnull可能な型を安全にアンラップします。
-
関数はどのように宣言されますか?なぜKotlin関数はトップレベル関数として知られていますか?
fun sumOf(a: Int, b: Int): Int{ return a + b }
:
の後に関数の戻り値の型が定義されます。Kotlinでは、関数はKotlinファイルのルートで宣言できます。 -
Kotlinで==と===演算子の違いは何ですか?
\== is used to compare the values are equal or not. === is used to check if the references are equal or not.
- public
- internal
- protected
- private
`public` is the default visibility modifier.
```
class A{
}
class B : A(){
}
```
**NO**. By default classes are final in Kotlin. To make them non-final, you need to add the `open` modifier.
```
open class A{
}
class B : A(){
}
```
Constructors in Kotlin are of two types: **Primary** - These are defined in the class headers. They cannot hold any logic. There's only one primary constructor per class. **Secondary** - They're defined in the class body. They must delegate to the primary constructor if it exists. They can hold logic. There can be more than one secondary constructors.
```
class User(name: String, isAdmin: Boolean){
constructor(name: String, isAdmin: Boolean, age: Int) :this(name, isAdmin)
{
this.age = age
}
}
```
`init` is the initialiser block in Kotlin. It's executed once the primary constructor is instantiated. If you invoke a secondary constructor, then it works after the primary one as it is composed in the chain.
String interpolation is used to evaluate string templates. We use the symbol $ to add variables inside a string.
```
val name = "Journaldev.com"
val desc = "$name now has Kotlin Interview Questions too. ${name.length}"
```
Using `{}` we can compute an expression too.
By default, the constructor arguments are `val` unless explicitly set to `var`.
**NO**. Unlike Java, in Kotlin, new isn't a keyword. We can instantiate a class in the following way:
```
class A
var a = A()
val new = A()
```
when is the equivalent of `switch` in `Kotlin`. The default statement in a when is represented using the else statement.
```
var num = 10
when (num) {
0..4 -> print("value is 0")
5 -> print("value is 5")
else -> {
print("value is in neither of the above.")
}
}
```
`when` statments have a default break statement in them.
In Java, to create a class that stores data, you need to set the variables, the getters and the setters, override the `toString()`, `hash()` and `copy()` functions. In Kotlin you just need to add the `data` keyword on the class and all of the above would automatically be created under the hood.
```
data class Book(var name: String, var authorName: String)
fun main(args: Array<String>) {
val book = Book("Kotlin Tutorials","Anupam")
}
```
Thus, data classes saves us with lot of code. It creates component functions such as `component1()`.. `componentN()` for each of the variables. [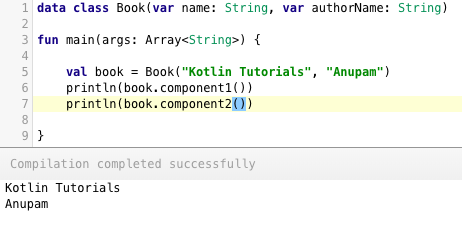](https://journaldev.nyc3.cdn.digitaloceanspaces.com/2018/04/kotlin-interview-questions-data-classes.png)
Destructuring Declarations is a smart way to assign multiple values to variables from data stored in objects/arrays. [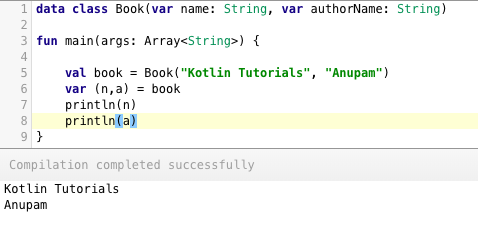](https://journaldev.nyc3.cdn.digitaloceanspaces.com/2018/04/kotlin-interview-questions-destructuring-declarations.png) Within paratheses, we've set the variable declarations. Under the hood, destructuring declarations create component functions for each of the class variables.
[Inline functions](/community/tutorials/kotlin-inline-function-reified) are used to save us memory overhead by preventing object allocations for the anonymous functions/lambda expressions called. Instead, it provides that functions body to the function that calls it at runtime. This increases the bytecode size slightly but saves us a lot of memory. [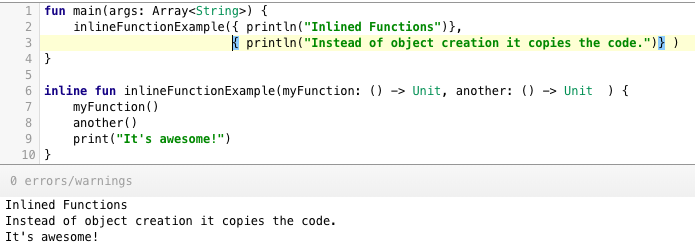](https://journaldev.nyc3.cdn.digitaloceanspaces.com/2018/04/kotlin-interview-questions-inline-functions.png) [infix functions](/community/tutorials/kotlin-functions) on the other are used to call functions without parentheses or brackets. Doing so, the code looks much more like a natural language. [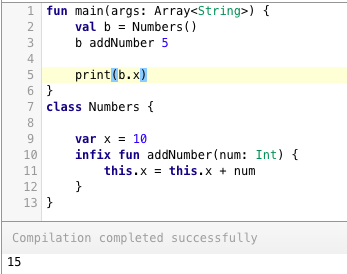](https://journaldev.nyc3.cdn.digitaloceanspaces.com/2018/04/kotlin-interview-questions-infix-notations.png)
Both are used to delay the property initializations in Kotlin `lateinit` is a modifier used with var and is used to set the value to the var at a later point. `lazy` is a method or rather say lambda expression. It's set on a val only. The val would be created at runtime when it's required.
```
val x: Int by lazy { 10 }
lateinit var y: String
```
To use the singleton pattern for our class we must use the keyword `object`
```
object MySingletonClass
```
An `object` cannot have a constructor set. We can use the init block inside it though.
**NO**. Kotlin doesn't have the static keyword. To create static method in our class we use the `companion object`. Following is the Java code:
```
class A {
public static int returnMe() { return 5; }
}
```
The equivalent Kotlin code would look like this:
```
class A {
companion object {
fun a() : Int = 5
}
}
```
To invoke this we simply do: `A.a()`.
```
val arr = arrayOf(1, 2, 3);
```
The type is Array<Int>.
Kotlinの面接の質問と回答は以上です。
Source:
https://www.digitalocean.com/community/tutorials/kotlin-interview-questions